Adventures in UPnP with node.js
Published 2015-2-7Please also see:
Find your Internet Gateway (router) with a UPnP Search Broadcast
This will
- Create a dgram socket bound to a random port
- Send an HTTP-like M-SEARCH message to discover an IGD (your router)
* message is sent to 239.255.255.250:1900 (hard-coded as per spec)
- Receives response from IGD from actual ip and dynamic port
* netcat would ignore such a response due to the ip mismatch
- Prints the message to the screen
- The
Location
field is what's important
UPnP SSDP Internet Gateway Device discovery example
wget https://raw.githubusercontent.com/coolaj86/holepunch/master/examples/upnp-ssdp-igd-discovery.js
'use strict';
var dgram = require('dgram')
, fs = require('fs')
, ssdpAddress = '239.255.255.250'
, ssdpPort = 1900
, sourceIface = '0.0.0.0' // or ip (i.e. '192.168.1.101', '10.0.1.2')
, sourcePort = 0 // chosen at random
, searchTarget = 'urn:schemas-upnp-org:device:InternetGatewayDevice:1'
, socket
;
function broadcastSsdp() {
var query
;
// described at bit.ly/1zjVJVW
query = new Buffer(
'M-SEARCH * HTTP/1.1\r\n'
+ 'HOST: ' + ssdpAddress + ':' + ssdpPort + '\r\n'
+ 'MAN: "ssdp:discover"\r\n'
+ 'MX: 1\r\n'
+ 'ST: ' + searchTarget + '\r\n'
+ '\r\n'
);
// Send query on each socket
socket.send(query, 0, query.length, ssdpPort, ssdpAddress);
}
function createSocket() {
socket = dgram.createSocket('udp4');
socket.on('listening', function () {
console.log('socket ready...');
broadcastSsdp();
});
socket.on('message', function (chunk, info) {
var message = chunk.toString();
console.log('[incoming] UDP message');
console.log(info);
console.log(message);
});
console.log('binding to', sourceIface + ':' + sourcePort);
socket.bind(sourcePort, sourceIface);
}
createSocket();
I've tested this against:
- Google Fiber NetworkBox
- Netgear AC ??? (TODO check model number)
- WRT54G-TM with DD-WRT
- WRT54GS with Linksys firmware
See nat-upnp for port-forwarding.
It will not work with
- Apple Airport Extreme
- Apple Airport Express
These use Zeroconf. If you know how to discover the ip address of an Apple router, I'd love to hear about it in the comments.
See nat-pmp for port-forwarding.
By AJ ONeal
Thanks!
It's really motivating to know that people like you are benefiting
from what I'm doing and want more of it. :)
Did I make your day?
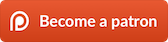
(you can learn about the bigger picture I'm working towards on my patreon page )