Getting Started with Golang on OS X
Published 2015-7-9- From 0 to Hello World
- Next steps
From 0 to Hello World
Let's say that you have brand new Macbook straight from the factory, here's what you need
- XCode Commandline Tools
- brew
- go
- $GOPATH
And here's how you get it:
XCode
Open up Terminal.app and copy and paste the following:
xcode-select --install
That will open up a window that asks you "are you sure blah blah blah". Just go with the affirmatives.
Once it's finished you should be able to use git
:
git --version
If that didn't work you may need to logout and log back in or restart
your computer. If it still doesn't work just try the xcode-select install
a second time.
brew
Next you need to get brew
(aka
Homebrew),
which is basically the open-source app store
for OS X (similar to apt-get
on Linux).
ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
That should only take a minute. You can (and should) test that it installed correctly:
brew doctor
Go
And finally you can install go
.
brew install go
$GOPATH
Before you can use go
you need to create and set your $GOPATH.
I like to put mine under my Code folder:
mkdir -p ~/Code/go
Then open ~/.bashrc
in your favorite text editor, or
vim,
and set $GOPATH.
vim ~/.bashrc
export GOPATH="${HOME}/go"
export PATH="${GOPATH}/bin:${PATH}"
Finally, you must refresh your current settings:
source ~/.bashrc
Great! Now you're ready to go!
(see what I did there?)
fish
If you use fish (and if you don't know, then you don't), you set yours like so:
vim ~/.config/fish/fish.config
set -gx GOPATH "$HOME/Code/go"
set -gx PATH "$GOPATH/bin" $PATH
Then refresh your current config
. ~/.config/fish/config.fish
Hello World!
Now we can make a little project to print "Hello, World!" to the console:
vim /tmp/hello.go
package main
import "fmt"
func main() {
fmt.Println("Hello, World!");
}
Then you can run it just like you would a script:
go run /tmp/hello.go
Or you can compile it and run it as a binary
go build -o /tmp/hello /tmp/hello.go
/tmp/hello
Next steps
- goimport
- gofmt
- godoc
- igo
You'll probably want to learn a little about setting up your development environment.
I have some info on getting go setup with vim in another article:
https://coolaj86.com/articles/today-i-became-a-golang-dev-with-vim-and-caddy/
It's a good idea to think of a small problem that you want to solve and then, instead of solving it the way you normally would, solve it in Go.
For example, I wanted a way to know whether or not a tcp port was already in use, so I created test-port in Go.
igo
It may be useful to you to have igo
to play with - either to test as a quick
way to test very basic go usage or just as a handy commandline calculator.
go get github.com/sbinet/igo
go build -o "$GOPATH/bin/igo" github.com/sbinet/igo
Then you can play around with primitives and math and such
igo
0.1 + 0.2
> 0.300000
buf = make([]byte, 10, 20)
len(buf)
> 10
cap(buf)
> 20
buf[0] = 1
append(buf, 0xff)
len(buf)
> 11
buf
{1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 255}
Unfortunately you can't use import, so it's not super useful, but it is kinda fun for tinkering.
Remember: ctrl+d
to exit. :-)
By AJ ONeal
Did I make your day?
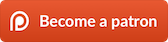
(you can learn about the bigger picture I'm working towards on my patreon page )