How does Angular's function argument black magic work?
Published 2013-11-22Watch on YouTube: youtu.be/Jyhz2e4grHo
I don't have much here in the way of written explanation, but it's explained pretty clearly in the video.
Also, you can check out @iammerrick's article on JavaScript Dependency Injection.
// Just an Ordinary Function
function say(what, to, from) {
console.log("Hey", to + "!"
, what + '!'
, '-' + from);
}
//
// Black Magic Explained
//
var argnames
, moduleMap
, args = []
, newFn
;
// Get the names of the arguments the function wants
// errata: in the video I had the regex slightly off
argnames = say.toString()
.replace(/\n/g, ' ')
.replace(/[^\(]+\(([^\)]*)\).*/g, '$1')
.split(/\s*,\s*/)
;
console.log(argnames);
// Create a hashmap of possible argument names
moduleMap =
{ to: "Bob"
, what: "Yo, wut up!?"
, from: "Alice"
, stranger: "Danger"
}
;
// Build the arguments to be passed from
// the hashmap, using the names as keys
argnames.forEach(function (argname) {
args.push(moduleMap[argname]);
});
// Use 'apply' to pass in the array as the 3 args
say.apply(null, args);
// If you wanted to do this on your own libraries you
// would need a build step that rewrote the file with
// literal string values before minification
// (and then use the keynames from the array instead)
newFn = '['
+ argnames.map(function (argname) {
return '"' + argname + '"';
}).join(', ') + ', '
+ say.toString()
+ ']';
console.log(newFn);
By AJ ONeal
Thanks!
It's really motivating to know that people like you are benefiting
from what I'm doing and want more of it. :)
Did I make your day?
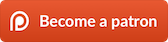
(you can learn about the bigger picture I'm working towards on my patreon page )