How to create a bookmarklet (and load jQuery anywhere)!
Published 2013-1-24Video not yet available
The Final Product
For those of you not as interested in the learning process, you can skip ahead to the final product: How to load jQuery anywhere.
Creating a Bookmarklet
Have you ever wanted to extend a website, but didn't know how? Have you experienced pain when migrating an extension originally developed for Firefox to Chrome? Are still reading this post?
If you answered yes to any of the questions of, bookmarklets may be right for you.
A bookmarklet is a bit of javascript that gets stored into a bookmark. When you click on the bookmark, it will run its javascript on whichever page you're currently on.
As an example, let's create a bookmarklet that loads jQuery onto a page.
First, however, let's just look at how to load jQuery "normally".
Linux and Windows users: Hit <ctrl> + <shift> + j
in Chrome.
That should open the JavaScript console.
Mac users: Hit ⌘ + ⌥ + j
(that's <command> + <option> + j
)
Copy and paste the following into the console (and then hit <enter>
):
var js = document.createElement('script')
;
js.src = "//ajax.googleapis.com/ajax/libs/jquery/1.9.0/jquery.min.js";
document.head.appendChild(js);
Note: you can check Google's jQuery CDN for the most up-to-date link (the thing that gets put as the src
),
as this post will eventually become out-of-date.
Congrats! Now do something crazy like replace the content of the page you're on with "Hello World!" (you do that this way):
$('body').text("Hello World!");
Well, that was stupid... now you've got no dom to hack with...
Create a folder ~/bookmarklet-demo
and go into it:
mkdir -p ~/bookmarklet-demo
pushd ~/bookmarklet-demo
Create a file index.jade
(use vim
) and put this in it:
Prerequistes:
- You know the basics of vim: vim for people who would rather not learn vim
- You have installed
node
: How to install NodeJS on Ubuntu - You have installed
jade
:npm install -g jade || sudo npm install -g jade
index.jade:
!!! 5
html
head
script(src="//ajax.googleapis.com/ajax/libs/jquery/1.9.0/jquery.min.js")
script(src="loader.js")
body
a.js-bookmarklet-link(href="#itstoosoon") jQuerify
Now compile that to html with jade index.jade
Now create these files:
loader.js
:
(function () {
"use strict";
var bookmarkletSource = "bookmarklet.js"
;
jQuery.get(bookmarkletSource, function (data) {
$('a.js-bookmarklet-link').attr(
'href'
, 'javascript:' + encodeURI(data.replace(/HOST/, window.location.host))
);
}, "text");
}());
bookmarklet.js
:
(function () {
"use strict";
var js
;
function getApp() {
var url = "http://" + "HOST" + "/bookmarklet-extra.js"
, js
;
js = document.createElement('script')
js.addEventListener('load', function () {
console.log("loaded the real deal");
});
js.src = url;
document.head.appendChild(js);
}
console.log('This bookmarklet begins!');
// this site may or may not have the jQuery we want...
// so we'll just get what we want and worry about the consequences
// if the happen!
js = document.createElement('script');
js.addEventListener('load', getApp);
js.src = "//ajax.googleapis.com/ajax/libs/jquery/1.9.0/jquery.min.js";
document.head.appendChild(js);
}());
bookmarklet-extra.js
:
(function () {
"use strict";
console.log("This bookmarklet is 7 kinds of boss!");
}());
Now serve it all up with python -m SimpleHTTPServer 3000
and visit
http://localhost:3000 to try it out.
-
Once the page loads open the console (as mentioned at the beginning).
-
Click on the jQuerify link (notice the output).
-
Now drag the jQuerify link to your bookmark bar (as seen here: http://youtu.be/5Hpw90i_Z7c )
-
Visit any page you like (maybe http://yahoo.com)
-
Open the console
-
Click the bookmarklet
-
Try this in the console:
$('body').text("pwn'd!");
Congratulations! You've just thrown cross-domain web security on the ground! 'cuz you're an adult!!!
Next steps:
Now that you have a basic template that can be used for any bookmarklet, here's how to make one that will add thumbnails to craigslist.
By AJ ONeal
Did I make your day?
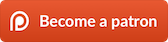
(you can learn about the bigger picture I'm working towards on my patreon page )