How to create and issue Trusted Peer SSL Certificates
Published 2015-3-20Here's the thing: End-to-end encryption is important.
That means that even inside your network you should encrypt traffic between machines. Don't pretend like visitors don't have access to your wifi. You know your employees give it out to all of their friends - and that's okay. It's not WiFi's job to protect your privacy. It's not ethernet's job to protect you. It's your job to protect you.
The solution is simple and easy to implement: use trusted-peer certificates.
I created the Node.js SSL Trusted Peer Example and I also wrote How to create a Certificate Signing Request (CSR) for HTTPS (TLS/SSL) RSA PEMs, but sometimes I just need a quick reference because as I add more services to my network, I need to repeat this process more and more often.
These examples are taken from Node.js SSL Example
Create the Certificate Authority
# make directories to work from
mkdir -p certs/{devices,client,ca,tmp}
# Create your very own Root Certificate Authority
openssl genrsa \
-out certs/ca/my-root-ca.key.pem \
2048
# Self-sign your Root Certificate Authority
# Since this is private, the details can be as bogus as you like
openssl req \
-x509 \
-new \
-nodes \
-key certs/ca/my-root-ca.key.pem \
-days 9131 \
-out certs/ca/my-root-ca.crt.pem \
-subj "/C=US/ST=Utah/L=Provo/O=ACME Signing Authority Inc/CN=example.com"
NOTE: Since I do these as one-off's I don't actually need to worry about the number of days the certificate is valid, so I set it for 25 years (assuming 19 non leap years and 6 leap years - just for fun). Hopefully this code won't exist by then.
Create and Sign the Peer Certificates
# Change to be whatever
FQDN='local.helloworld3000.com'
DEVICE_NAME='my-device'
# make directories to work from
mkdir -p certs/{devices,client,ca,tmp}
# Create Certificate for this domain,
openssl genrsa \
-out certs/devices/${DEVICE_NAME}.key.pem \
2048
# Create the CSR
openssl req -new \
-key certs/devices/${DEVICE_NAME}.key.pem \
-out certs/tmp/${DEVICE_NAME}.csr.pem \
-subj "/C=US/ST=Utah/L=Provo/O=ACME Service/CN=${FQDN}"
NOTE: It is bad practice to create all of the keys and sign them on the same machine, but the machine I use to create the certs is my own and once I've deployed the certs to the peers, I destroy the folder, so I'm not worried about it.
Sign the Certs
DEVICE_NAME='my-device'
# Sign the request from Device with your Root CA
openssl x509 \
-req -in certs/tmp/${DEVICE_NAME}.csr.pem \
-CA certs/ca/my-root-ca.crt.pem \
-CAkey certs/ca/my-root-ca.key.pem \
-CAcreateserial \
-out certs/devices/${DEVICE_NAME}.crt.pem \
-days 9131
# If you already have a serial file, you would use that (in place of CAcreateserial)
# -CAserial certs/ca/my-root-ca.srl
Deploy the Certs
DEVICE_HOSTNAME='my-device.mynetwork.com'
DEVICE_NAME='my-device'
rsync -avhHPz certs/ca/my-root-ca.crt.pem "${DEVICE_HOSTNAME}:/srv/certs/ca/"
rsync -avhHPz certs/devices/${DEVICE_NAME}.* "${DEVICE_HOSTNAME}:/srv/certs/server/"
rm -rf "certs/devices/${DEVICE_NAME}.*"
Wrapping Up
If I wanted to I could go ahead and delete the root ca's key at this point.
By AJ ONeal
Did I make your day?
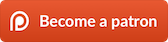
(you can learn about the bigger picture I'm working towards on my patreon page )