How to pick a facebook fan at random
Published 2013-4-16Intuitively you would think there would be an API call to get a paginated list of fans, but our peers at StackOverflow haven't yet discovered it, so I doubt we will either.
However, assuming you don't have 63 million fans, like Coca Cola does, there are a few ways to find them.
Option A: Use FacePile (the fan iFrame)
Let's say your page is something like https://www.facebook.com/CoolAJtheDJ.
You can replace the www
with graph
-
as in https://graph.facebook.com/CoolAJtheDJ -
and the object you get back will have the id
.
If you we're talking somewhere in the range of 100s of fans, you could retrieve the public fan list, scrape it a few times, and track the uinque fans.
If all you're looking to do is to get a single random fan, you can just pick the very last in this list and that will suffice. (the first seems to always be the same)
https://www.facebook.com/plugins/fan.php?connections=100&id=136573516451237
Note: You must visit that page as a user, not as a page.
(Hurrah for incognito mode! Ctrl + Shift + N
)
var allTheFans = [].slice.call( $$('.pluginFacepile li a') );
console.log(allTheFans[0].title, allTheFans[0].href);
Recap
- https://www.facebook.com/CoolAJtheDJ
- https://graph.facebook.com/CoolAJtheDJ
- https://www.facebook.com/136573516451237
- https://www.facebook.com/plugins/fan.php?connections=100&id=136573516451237
Option B: Use 'Likes' on Page Insights
Go to https://www.facebook.com/CoolAJtheDJ/page_insights_likes (replacing 'CoolAJtheDJ' with your own page name) and then click "See Likes" and then scroll 'til it won't scroll no more.
Note: In Chrome $$
is an alias of document.querySelectorAll
,
either of which we can use to get the fans
(and turn the DOM NodeList into a normal JavaScript Array using [].slice.call()
as a hack).
var allTheFans = [].slice.call( $$('li.fbProfileBrowserListItem a[gt-data]') );
console.log(allTheFans[0].innerText, allTheFans[0].href);
The nice thing about this approach is that you know the most recent fans are on top and you can gather just the most recent fans each week (or the last time since you ran the contest), if that's what you're looking for.
Shuffle and Choose a Winner
Check out http://bost.ocks.org/mike/shuffle/ for an explanation of how Math.random() is broken.
function shuffle(array) {
var m = array.length, t, i;
// While there remain elements to shuffle…
while (m) {
// Pick a remaining element…
i = Math.floor(Math.random() * m--);
// And swap it with the current element.
t = array[m];
array[m] = array[i];
array[i] = t;
}
return array;
}
var winningFan = shuffle(allTheFans)[0];
console.log(winningFan.innerText || winningFan.title, winningFan.href);
And now I can contact this person and let them know that they have won!
... now how do you contact someone from a fan page?
By AJ ONeal
Did I make your day?
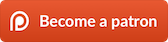
(you can learn about the bigger picture I'm working towards on my patreon page )