How to install Ruby (and RVM) on Ubuntu (for technotards)
Published 2013-1-18Ruby what?
ruby
is a programming language.
Almost every static blog generator -
ruhoh, octopress, jekyll, nanoc, etc -
is written in ruby.
A lot of web apps (such as the original twitter)
and system utilities are written in ruby as well.
Ruby is probably the easiest to learn of all the programming languages. Check out http://tryruby.org when you have 15 minutes to spare.
RVM and Bundler What?
Among the many great things that ruby has done for the world, it brought us ruby gems (versioned ruby modules). gems make ruby programming really really fast because you can usually find a gem that already solves your problem (such as parsing JSON or logging in through facebook) much faster than you can read the documentation or specification (or otherwise figure out how) to solve the problem yourself. Also, the language itself is amazingly simple to work with.
As an unfortunate side effect of people making programs in record time, many modules were iterated over several versions in short time. Thus was born version hell.
version hell is the state of confusion encountered by mere mortals during which they are unable to figure out how to install an app or module because one of the modules it depends on was updated to a newer version which is not compatible with another app or module which you are also using.
rvm
(ruby version manager)
and
bundler
(bundles ruby gems for a single app or module,
rather than installing them globally on your system),
along with the semver specification,
were created to vanquish version hell.
Let's install Ruby!
Can we install it? Yes we can!
Removing Ubuntu's old rvm and ruby
Ironically, Ubuntu's desktop edition ships with a version of rvm that is... a wrong version.
If it's installed and you leave it, your Ubuntu will become sad when you try to install the real rvm.
It's not a big deal, but we do need to get rid of it from the system before we start.
sudo apt-get remove --purge ruby-rvm ruby
sudo rm -rf /usr/share/ruby-rvm /etc/rmvrc /etc/profile.d/rvm.sh
It will also probably be useful to get rid of any rvm bits laying around your user folder as well (you may not have any, and that's okay).
# See if there is anything
ls -d ~/.rvm*
ls -d ~/.gem*
ls -d ~/.bundle*
# Delete them (ignoring those that don't exist)
rm -rf ~/.rvm* ~/.gem/ ~/.bundle*
Note: #
is a comment marker.
Everything after it is ignored by the terminal.
It's just there for your reading pleasure.
Once you've done that, <ctrl>+d
to exit out of your terminal. Then open a new one.
Not installing rdoc and ri
Many ruby modules install documentation in rdoc
and ri
format
and it makes the install go so much slower.
To install modules without documentation by default,
you can append gem: --no-rdoc --no-ri
to the file .gemrc
in your home folder. The fastest way to do this is to
use echo
(print to output) with the >>
(redirect output to append to end of file) operator.
You can then double check by using tail
which is like cat
, but only outputs the last few lines of the file
instead of the whole thing.
echo 'gem: --no-rdoc --no-ri' >> ~/.gemrc
tail ~/.gemrc
You should see gem: --no-rdoc --no-ri
printed out as the contents of
the file (possibly with other output before it)
Alternatively, you could vim ~/.gemrc
and then add the line
gem: --no-rdoc --no-ri
on any line of the file.
If you inspect .gemrc
(i.e. using vim
) and
find that that line is repeated more than once, delete the extra lines.
There's no harm in having it in there extra times,
but there's no benefit to it either.
Installing via rvm
First we'll make sure that your system has all the prerequisite system utilities. There's no harm in doing this gain if you've done it before. It will simply skip whatever has been installed previously.
sudo apt-get update
sudo apt-get install -y \
git \
build-essential \
curl \
wget
Note: When you are copy-pasting commands and a line ends with \
,
you must copy all of the following lines up until the last one that does not have a **.
Usually the lines will be indented as you see above.
In this case the indentation doesn't mean anything to the computer,
it's just a cue to the reader.
Note: For some stupid reason, apt-get
will often ask you something
to the affect of are you sure? whenever an installation is more than
a few killobytes in size.
Effectively -y
(same as --yes
) tells apt-get
shut up and do what I say unless there's real problem.
Next we'll add a script that tells bash
and zsh
to load rvm at
each login.
# For bash
echo "[[ -s '${HOME}/.rvm/scripts/rvm' ]] && source '${HOME}/.rvm/scripts/rvm'" >> ~/.bashrc
# For zsh
echo "[[ -s '${HOME}/.rvm/scripts/rvm' ]] && source '${HOME}/.rvm/scripts/rvm'" >> ~/.zshrc
Note: The &&
is a way to say run this next command, but only if the previous command had no errors.
Now for the meat of the issue, installing rvm:
curl -L https://get.rvm.io | bash -s stable
There's a lot going on in that one little line. Essentially it's saying Hey curl
, go get http://get.rvm.io and hand it off to bash
which will run it as a script with the -s stable
argument... whatever that means.
IMPORTANT NOTE: You'll might see a notice telling you to install about a brazillion things,
but I recommend not installing sqlite3
, libsqlite3
, or libsqlite3-dev
,
because the Ubuntu repository is out of date and lacks SQLite3-FTS support.
See github.com/coolaj86/node-sqlite-fts-demo for instructions on
installing sqlite3
and co. with FTS support.
Oh, and ignore any warnings about .profile
Anyway, to install the approximately 3 brazillion things it needs (and may or may not ask you about), copy and paste the whole block of the following into the terminal:
sudo apt-get install -y \
build-essential \
openssl \
libreadline6 \
libreadline6-dev \
curl \
git-core \
zlib1g \
zlib1g-dev \
libssl-dev \
libyaml-dev \
libxml2-dev \
libxslt-dev \
autoconf \
libc6-dev \
ncurses-dev \
automake \
libtool \
bison \
subversion \
pkg-config
And again, either you need to follow the sqlite-fts installation instructions or install non-fts sqlite with the following:
sudo apt-get install -y \
sqlite3 \
libsqlite3-dev
And then run the rvm installer again, but this time we'll add --ruby
to tell it that we are, in fact, prepared to install ruby in all of its
glory.
curl -L https://get.rvm.io | bash -s stable --ruby
That will take a while to run... maybe 10 minutes. Time for a coffee break!
Now test that the installation was successful by loading ruby:
source ~/.rvm/scripts/rvm
rvm reload
rvm use default
ruby --version
cyaml
isn't installed by default, but you'll need it (I promise), so install it now.
gem install cyaml --no-ri --no-rdoc
If you skipped installing sqlite3
from source and didn't install it from apt-get either,
when you do get around to installing it
you'll nee to run.
bash -c "rvm reinstall 1.9.3"
IMPORTANT NOTE: For compiling and installing, bash
seems to be more successful than zsh
.
Once installed, zsh
should work just as well for running ruby.
Install bundler
For whatever reason, bundler doesn't get installed with rvm by default.
Install it like so:
gem install bundler --no-ri --no-rdoc
Ruby Playground
If you want to play around with ruby you can enter the "ruby shell"
(so-to-speak). Enter irb
(interactive ruby) into the terminal.
irb
When you're done (or completely lost) hit <ctrl>+d
to exit back to your normal shell.
Appendix
Installing via apt-get
Ruby used to be a very fast-moving target (kinda like how NodeJS is today), but it's slowed down enough that the Ubuntu repositories have a pretty recent version that's probably good enough.
If you were successful with the method above, don't do this!
If you find later on that you were unsuccessful in installing ruby, and bundler in a way that works for your project using the method above, give this a try:
rm -rf ~/.bundle ~/.gem ~/.rvm
sudo apt-get update
sudo apt-get install ruby1.9.3
sudo apt-get install \
libxslt1-dev \
libxml2-dev \
libyaml-dev
sudo gem install cyaml --no-ri --no-rdoc
sudo gem install bundler --no-ri --no-rdoc
Resources
- INSTALL RVM ( RUBY VERSION MANAGER ) IN UBUNTU 12.04 LINUX FOR RUBY 1.9.3
- http://stackoverflow.com/questions/9056008/installed-ruby-1-9-3-with-rvm-but-command-line-doesnt-show-ruby-v/9056395
By AJ ONeal
Did I make your day?
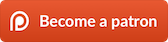
(you can learn about the bigger picture I'm working towards on my patreon page )