Introduction to HTTP with Netcat, Chrome, NodeJS, and Connect (ExpressJS)
Published 2013-3-12Watch on YouTube: youtu.be/0CZyV3ghZAg
Here's to day 2 or so of Zero Day Web Development.
It's high time you took a raw hard look at HTTP and learned to use real tools like netcat and Chrome's network debugger. After that, it's time to programatically recreate the experience using node and then connect (which is at the heart of expressjs).
Networking with Ping and Netcat
- use
ifconfig
to find the IP address ofen0
,en1
,wlan0
, oreth0
ping -c 1
the IP address of the other computer to test connectivitynetcat -l 4000
on one computer to listennetcat
to the ip and port from the other computer- You can chat back and forth
Viewing HTTP with Chrome, Netcat and NodeJS
-
With the netcat listener open Chrome to http://localhost:4000
-
view the message sent by Chrome
-
respond with a valid HTTP response by hand
HTTP/1.1 200 OK Content-Type: text/plain; charset=utf-8 Content-Length: 4 Hi!
-
Now build a node http server to handle the response, but with the message "Hello World!"
// test-http.js // See docs at http://nodejs.org/api/http.html (function () { "use strict"; var http = require('http') , port = 4000 , server ; function handleAllRequests(request, response) { console.log(request.method, request.url, request.httpVersion); console.log(request.headers); response.setHeader('Content-Type', 'text/plain; charset=utf-8'); response.write('Hello World!'); response.end(); // TODO inspect sentHeaders } function onListening() { console.log('Server listening on', server.address()); } server = http.createServer(handleAllRequests); server.listen(port, onListening); }());
-
Refresh the browser at http://localhost:4000 to see the change.
-
Also inspect the headers in the dev console.
Building a Web stack with Connect
-
Fix permissions if necessary
chown -R `whomai` ~/.npm/ ~/tmp/ sudo chown -R `whomai` /usr/local/
-
Install connect via
npm install connect
-
Simplify the server so that various modules can handle individual tasks via connect
// test-connect.js // See docs at http://www.senchalabs.org/connect/ (function () { "use strict"; var connect = require('connect') , port = 4000 , server ; function logger(req, res, next) { console.log(request.method, request.url, request.httpVersion); console.log(request.headers); next(); } function handleHelloRequests(request, response) { response.setHeader('Content-Type', 'text/plain; charset=utf-8'); response.write('Hello World!'); response.end(); // TODO inspect sentHeaders } function onListening() { console.log('Server listening on', server.address()); } app = http.createServer(); app.use(logger); app.use(connect.favicon()); app.use(connect.static(__dirname)); app.use('/hello', handleHelloRequests); server = app.listen(port, onListening); }());
-
Request each of the following
By AJ ONeal
Did I make your day?
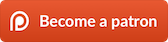
(you can learn about the bigger picture I'm working towards on my patreon page )