memcpy SIGBUS (duh)
Published 2011-1-24I just had a #FFD700
moment.
A quick Google of memcpy SIGBUS
lead me on a wild goose chase about alignment errors.
However, the problem for me was an extremely simple one - the kind that you might expect -Wall -Werror
should catch.
int main(int argc, char* argv[])
{
char* str = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ"; // 36 + 1
memcpy(str, "abcdef", 3);
printf("%s\n", str);
// abcdef6789...
return 0;
}
What's the issue here? Well, str
is actually a const char*
because it's loaded into read-only memory!
int main(int argc, char* argv[])
{
const char* str_const = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ"; // 36 + 1
char* str = malloc(strlen(str_const) + 1);
memcpy(str, str_const, strlen(str_const) + 1);
memcpy(str, "abcdef", 3);
printf("%s\n", str);
// abcdef6789...
return 0;
}
By AJ ONeal
Thanks!
It's really motivating to know that people like you are benefiting
from what I'm doing and want more of it. :)
Did I make your day?
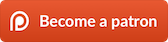
(you can learn about the bigger picture I'm working towards on my patreon page )