Weak Timeout and Event Loop References
Published 2020-3-31A node will keep running as long as there is a strong reference in the Event Loop.
Sometimes you need to have something happen on a timer, but you don't want that timer to keep the process alive.
For that you can use unref()
:
setTimeout(fn, ms).unref();
unref()
all the things
Other than setTimeout
and setInterval
, there are a number of Node APIs that support unref()
to create weak references in the event loop.
This can be useful for servers, database connections, child processes, and many other things.
setTimeout(fn, ms).unref();
setInterval(fn, ms).unref();
setImmediate(fn).unref();
child_process.fork(modulePath).unref();
child_process.spawn(command).unref();
net.createServer(options).unref();
http.createServer(options).unref();
Docs:
- https://nodejs.org/api/timers.html#timers_timeout_unref
- https://nodejs.org/api/net.html#net_server_unref
- https://nodejs.org/api/child_process.html#child_process_subprocess_unref
If you're using the serverless
framework for lambda functions or some such,
you can set callbackWaitsForEmptyEventLoop = false
to work around strong references.
Example
function weakBackgroundTask() {
console.log('interval weak reference (50ms)');
}
function backgroundTask() {
console.log('releasing strong reference');
// program will exit now
}
console.log('creating an interval weak reference (every 50ms)');
setInterval(weakBackgroundTask, 50).unref();
console.log('creating a one-time strong reference (in 500ms)');
setTimeout(backgroundTask, 500);
References
By AJ ONeal
Thanks!
It's really motivating to know that people like you are benefiting
from what I'm doing and want more of it. :)
Did I make your day?
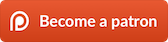
(you can learn about the bigger picture I'm working towards on my patreon page )