Things ES6 should have fixed...
Published 2015-6-3I said this
ES5 was a really good idea: remove the bad parts. ES6/ES7 are just spoon-feeding us ES4: more complexity / bugs #whereareyoucrockford
Which lead to this
+DouglasCrockfordEsq Why is ES6 growing into an unstoppable monster of complicated mess? I miss the mentality of ES5: fix the broken crap, add conveniences for novice users to make it easier to code without mistakes.
And then this
The Web Platform Podcast #43: Modern JavaScript with ES6 & ES7,
And now I want to take a moment to immortalize them on my own blog...
Feels Like We Only Go Backwards
Back in 2012 technology was truly magical. There was a moment when I really thought that we had all the bases covered and it would just upwards and onwards without delay. The future seemed so bright...
And then this happened:
- Apple shipped the last-ever MacBook with user-serviceable parts
- Sublte Gradients were trambled by Flat Design
- All UX-related buzzwords disappeared
- I stopped dating a pretty good girl for no good reason
- CoffeScript was renamed ES6
- ES6 was renamed ES2015
In summary: Life started to suck and I blame it all on ES6!
Music to write ES6 classes to: https://youtu.be/wycjnCCgUes
I think I even felt a great disturbance in the force as if millions of well-established best practices and good ideas cried out at once and were suddenly silenced.
I don't know for sure what happened, but I have my guesses.
JavaScript: The Missing Parts
Here are some of my biggest beefs with JavaScript as-is - now I can understand that it's no necessarily ES6's job to fix all of these problems, but it definitely should have tried to fix some of them, especially ones that cause community fallout - i.e. because the fixes require hundreds or thousands of lines of code that everyone feels differently about.
Make functions composable
Due to callbacks, composing h(g(f(x)))
is broken.
This is a programming language. If composing requires knowledge of internal implementation details, it's flat-out busted.
Promises effectively solve this. Yay!
Unicode, UTF-8, TypedArrays, Base64, and Hex
We got TypedArray
s and they work great.
However, we didn't get any utilities to convert to and from a simple string... what?!?
I can compose a gzipped tar file with a binary image, but I can't convert from a string to a buffer... how did node get this so right, but browsers and ES6 got it so wrong?
Fix the Maths
It's a problem.
0.1 + 0.2 === 0.30000000000000004
I don't know how you go about fixing it, but since most of the media APIs have values between 0 and 1 it really sucks when you spend a few hours trying to figure out why this doesn't work:
if (0 !== audio.volume) {
audio.volume -= 0.1;
}
Not just that, but did you know you can almost write device drivers in JavaScript?
We can or x |= 0xAF
, bit shift x << 2
, and even triple bit shift x <<< 2
.
The only problem is that most registers are 64- or 32-bits and sadly the 31-bits
JavaScript decidates to bitwise operations isn't a multpile of either of those...
0xffffffff !== 0xffffffff | 1
// fail
Streams / Emitters / Promitters
Promises solve one type of problem very well. Streams solve a different type of problem very well.
Standardizing an Emitter or Stream and probably a combo "Promitter" would have been great.
Standardize the things that exist everywhere anyway
I realize that setTimeout
isn't technically part of JavaScript, but it should be.
It's implemented in every environment.
If there's any environment where it isn't implemented, they're DEFINITELY doing it wrong.
- setTimeout
- crypto
- console
You can tell me until you're blue in the face that "it's up to the vender". If every vendor is going to have to implement it, it's better that there's a standard implementation that is perhaps less-than-perfect than that every vender has a nearly-compatible verison.
Throw ALL THE ERRORS
Things that take a limited set of correct inputs shouldn't error to succeeding with a default value, they should error out!
setTimeout(doStuff, 'muffins')
- that's a fail.
Existance and Prototypes
Here's one for the wishlist because it may add complexity and I haven't really thought it through, but it would be awesome if you could do stuff like this:
var foo = null;
var str = foo?.thing?.subthing?.toString(); // ""
instead of
var foo = null;
var str = "";
if (foo && foo.thing && foo.thing.subthing) {
str = foo.thing.subthing.toString();
}
Prevent Typos with Progressive Typing
I just want some standard syntax to annotate "here's what I expect this object to look like". I don't necessarily want big heavy classes with lots of syntax, especially not new syntax, just a simple way to progressively enhance my code.
I would imagine two new optional modes, maybe something like 'use transitional';
(warn in the console) 'use typed';
(throw an error).
Encourage Serializable / Deserializable patterns
I don't know how this would be implemented, it's just on my wishlist.
So many times devs get fancy with classes and prototypes and whatever and then I end up with this object that I really want to transmit and reconstitute on the server or send to a peer via WebRTC or put into another tab, but to do so I have to either write my own version of the module or write really hacky toJSON and fromJSON functions.
ES6: The Bogus Parts
Here's a legitimate problem that ES6 fixed:
- Promises
- Standard Module System (though I'm not convinced
import
is the answer)
Here's are some legitimate problem that ES6 created:
- Entirely new syntax for everything based on CoffeeScript
- More ways to misunderstand variables a la
let
- New ways to misunderstand async via
async
- Yet another competing module system, but one that can't polyfill
CoffeeScript & TypeScript
90% ES6 seems to be syntax sugar. It's just CoffeeScript.
But we didn't need anyone to formalize CoffeeScript in order to have Babel and Traceur - we already had CoffeeScript!
Another 9% of it seems to be TypeScript.
The last 1% is Promises and two or three other useful features.
I'm not saying TypeScript and CoffeScript are bad, I'm just saying that they don't belong in JavaScript.
WeakMap, WeakSet... what?
var map = {};
map[id] = person;
var set = {};
if (!set[value]) {
set[value] = true;
}
What was so hard about that?
I HATE BUILD TOOLS
ES6/7/8 will never exist, not because browsers won't implement them, but because there will never be a post-ES5 generation of developers that actually write JavaScript.
From now on there will always be proposed new features that are "just around the corner" that can't be implemented with a polyfill.
The transpilers are here to stay and since the elite have to use them to get their hands in the goodie bags, they'll always be reaching in, never satisfied.
What's a point of a dynamic language if I have to compile it to use it?
... try developing on a raspberry pi sometime. You might find the build step that takes "just a few seconds" takes quite a bit longer.
Too many legos (that fit both ways)
The biggest problem with JavaScript is that there's no instruction manual - and the vast majority of the online guides are written by people who are just learning themselves and admittedly have no real idea what they're doing.
It's the blind leading the blind (the same as PHP).
We were finally getting to a stage where ES5 best practices were being adopted -
'use strict';
has become ubiquitous, for example - and now we're dumping a whole
load of new tools that no one knows how to use and it's just going to create
more confusion.
It's better to just have Promises, which can be polyfilled, and not add new language constructs that change our understanding of async code.
const
I'm okay with. It adds a constraint that is easy to understand and
that will throw an error when used improperly. I understand that the ninja
devs want let
for performance, but from the last argument I heard I would
guess that allowing a const function
that couldn't have its bindings and
scope dynamically swept out from under it would solve that problem (throw
an error if you do a bad thing) instead of introducing complexity.
Chrome got one thing right
Not part of ES6, but I love what Chrome did with WebCrypto.
If you try to use it on an http
domain instead of https
, it throws an error.
That's mode programming at its finest.
We need more of that - require that the developer accept new constraints in order to use new features.
By AJ ONeal
Did I make your day?
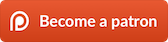
(you can learn about the bigger picture I'm working towards on my patreon page )