Convert a TypedArray Buffer to Base64 in JavaScript
Published 2015-6-26See also
- Unicode, UTF-8, TypeArray, Uint8Array, JavaScript, and You
- Direct Unicode to Uint8Array JavaScript conversion
This code was inspired by Unibabel JS, which in turn was inspired by MDN's The Unicode Problem.
If you need a pure "vanilla" JavaScript implementation which does not rely on the DOM or node.js, check out beatgammit's base64-js.
Note: You can get this to work all the way back to MSIE6 by replacing 'Uint8Array()' with 'Array()' and providing an Array polyfill for 'map' and 'forEach'.
from Uint8Array to Base64
'use strict';
function bufferToBase64(buf) {
var binstr = Array.prototype.map.call(buf, function (ch) {
return String.fromCharCode(ch);
}).join('');
return btoa(binstr);
}
Demo
// "I ½ ♥ 💩";
var arr = [73, 32, 194, 189, 32, 226, 153, 165, 32, 240, 159, 146, 169];
var data = new Uint8Array(arr);
var base64 = bufferToBase64(data); // "SSDCvSDimaUg8J+SqQ=="
from Base64 to Uint8Array
'use strict';
function base64ToBuffer(base64) {
var binstr = atob(base64);
var buf = new Uint8Array(binstr.length);
Array.prototype.forEach.call(binstr, function (ch, i) {
buf[i] = ch.charCodeAt(0);
});
return buf;
}
Demo
var base64 = "SSDCvSDimaUg8J+SqQ==";
var buf = base64ToBuffer(base64);
var arr = Array.prototype.slice.call(buf);
// "I ½ ♥ 💩";
// [73, 32, 194, 189, 32, 226, 153, 165, 32, 240, 159, 146, 169]
This needs to be standardized!
Please contact your local JavaScript standardization committee to recommend that a feature be standardized in JavaScript.
Note: That atob
and btoa
are not even part of JavaScript, they are proprietary to the DOM.
By AJ ONeal
Thanks!
It's really motivating to know that people like you are benefiting
from what I'm doing and want more of it. :)
Did I make your day?
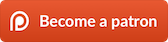
(you can learn about the bigger picture I'm working towards on my patreon page )