Getting Started with Golang and Vim
Published 2015-7-10Watch on YouTube: youtu.be/Gen2OXG-3f8
Things we need to talk about.
- vim-go
- gofmt
- goimport
- golint
- gocode
- godef
Other great resources
Rant/Rave
Go is beautiful in that it doesn't leave any room for bickering, bikeshiding, or otherwise b... complaining about stupid stuff that doesn't matter - like spacing, tabs, semicolons, etc.
If you're going to contribute code (or write code) without looking like an
idiot, you need to have gofmt
run everytime you save a Go file so that
it will automatically convert your spaces and such.
Unfortunately, vim isn't so lucky. There used to be one standard plugin
manager for vim: pathogen
. Now there are 3 or 4 of them and there's
nothing really different about them except that using one or the other
will make you feel more or less elitist than someone who has a stronger
or weaker opinion than you that's different from yours.
Because I'm not an elitist and I haven't seen any technical or cultural
advantage to the other loaders, we're still going to use pathogen
,
which works awesomely.
Is Go correctly installed?
Try these and see what you get
go version
> go version go1.4.2 darwin/amd64
And also
echo $GOPATH
> /Users/aj/Code/go
If either of those don't work, please see Getting Started with Go before going any further.
Do you have the latest vim?
If you're not too familiar with vim yet, I'd also recommend Vim for people who would rather not use Vim.
Don't use the version bundled with OS X.
I honestly don't remember why, but I remember some tool I was using needed features from the newest version (which is super-easy to install).
brew update
brew install vim
> vim-7.4.712
If you get an error about not having brew just run this (and then go back and rerun that):
ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
You're Ready for vim + Go!
You will need the generic vim-for-coders modules and then a few go specific tweaks.
generic vim setup
- pathogen
- vim-sensible
- syntastic
To have a "this doesn't suck" experience with vim you need to install
pathogen
(the vim package manager), so that you can install vim-sensible
.
To have a "this is awesome" experience with vim you need to install
syntastic
(code highlighting and is-it-broke checker tool) to start with,
and you'll add other stuff specific to language xyz and whatnot as you go.
So, from the top, in case you haven't done this before:
# Install pathogen
mkdir -p ~/.vim/autoload ~/.vim/bundle && \
curl -LSso ~/.vim/autoload/pathogen.vim https://tpo.pe/pathogen.vim
# Install vim-sensible
git clone git://github.com/tpope/vim-sensible.git ~/.vim/bundle/vim-sensible
# Install syntastic
git clone https://github.com/scrooloose/syntastic.git ~/.vim/bundle/syntastic
Now you need to turn pathogen
, which enables all of the other modules to
load automatically. You'll also need on and tell syntastic
vim ~/.vimrc
" plugins expect bash - not fish, zsh, etc
set shell=bash
" which key should be the <leader>
" (\ is the default, but ',' is more common, and easier to reach)
let mapleader=","
" pathogen will load the other modules
execute pathogen#infect()
" we want to tell the syntastic module when to run
" we want to see code highlighting and checks when we open a file
" but we don't care so much that it reruns when we close the file
let g:syntastic_check_on_open = 1
let g:syntastic_check_on_wq = 0
" we also want to get rid of accidental trailing whitespace on save
autocmd BufWritePre * :%s/\s\+$//e
" tell vim to allow you to copy between files, remember your cursor
" position and other little nice things like that
set viminfo='100,\"2500,:200,%,n~/.viminfo
Go Stuff
Now there are tons of cool things you can do and add and whatnot, but I'm a lame-O, so I'm just presenting you with the most common ones that 90% of people use, not the esoteric, elite, or really fun ones.
First you just need to install go tools that aren't included in the standard install, but are super convenient:
# goimports
go get -u golang.org/x/tools/cmd/goimports
# gocode
go get -u github.com/nsf/gocode
# godef
go get -u github.com/rogpeppe/godef
# golint
go get -u github.com/golang/lint/golint
# errcheck
go get -u github.com/kisielk/errcheck
# oracle
go get -u golang.org/x/tools/cmd/oracle
All of them automatically install to $GOPATH/bin/
,
which is /Users/aj/Code/go/bin/
in my case.
If any of them didn't install there you could manually put them there
with go build
- like this:
go build -o "$GOPATH/bin/" golang.org/x/tools/cmd/goimports
Tying vim + Go together
vim-go is the module that ties vim and go and all those go tools together is perfect unity.
You install it like so:
# Install vim-go
git clone https://github.com/fatih/vim-go.git ~/.vim/bundle/vim-go
# Install YouCompleteMe (requires building)
git clone https://github.com/Valloric/YouCompleteMe.git ~/.vim/bundle/YouCompleteMe
# the build step
brew install cmake
pushd ~/.vim/bundle/YouCompleteMe
git submodule update --init --recursive
./install.sh
popd
And then you need to make a minor edit to your vim config to tell vim-go
that you have, in fact, installed goimports
.
You can add this to the bottom of the file - or anywhere as long as it
comes after execute pathogen#infect
.
vim ~/.vimrc
" use goimports for formatting
let g:go_fmt_command = "goimports"
" turn highlighting on
let g:go_highlight_functions = 1
let g:go_highlight_methods = 1
let g:go_highlight_structs = 1
let g:go_highlight_operators = 1
let g:go_highlight_build_constraints = 1
let g:syntastic_go_checkers = ['go', 'golint', 'errcheck']
" Open go doc in vertical window, horizontal, or tab
au Filetype go nnoremap <leader>v :vsp <CR>:exe "GoDef" <CR>
au Filetype go nnoremap <leader>s :sp <CR>:exe "GoDef"<CR>
au Filetype go nnoremap <leader>t :tab split <CR>:exe "GoDef"<CR>
Celebrate
That's about it. Nothing too fancy and now you code in golang faster and you can contribute to go code without looking like a noob.
So SLOW!!!
If you find that vim begins to crawl you can always delete plugins
from ~/.vim/bundle/
and and limit the number of syntax checkers
in ~/.vimrc
.
rm -rf ~/.vim/bundle/YouCompleteMe/
let g:syntastic_go_checkers = ['golint']
See Also
There are some more tips and tricks from the vim-go author over at Gopher Academy: Go development environment for Vim
And also check out Programming with go in Vim.
Things you might like to google to learn next
- oracle
- gotags
- ctrlp
- nerdtree
By AJ ONeal
Did I make your day?
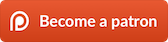
(you can learn about the bigger picture I'm working towards on my patreon page )